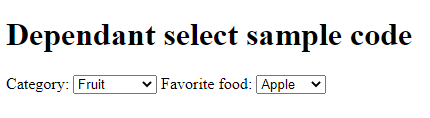
Select dependant on other select
The need for this solution pops up just rarely enough so I have time to forget where I wrote some similar code last time, and there are always some minor details I have to lookup in order to get everything to work properly. This time I decided to put it in my blog. That way I can always look it up later.
First we need to setup the html. One category select. It represent the trigger that should update the second select, in this case our food select. The first time I did this, I put all the options in the food select, looped through them and added display: none; or something similar to the once I did not want to be visible. But a few weeks later the customer came back and asked why the filter had stopped working... I did not do anything and as I tested the code still worked... Turned out our customer hade just started using internet explorer where you, at least at that point, could not hide options in a select that way. That is why we add a second select I call food_resource. Sometimes I have the resources in a div containing a json_object that I just can read and work with. In some cases that does make more sence, but in this instance I wanted to just copy the relevant options from a hidden select over to the visible one.
Category:
Favorite food:
Over to the javascript. Since the food select is empty when the page is loaded the first thing that happends is that the function updateFood(); is called. It start with cleaning out any options from the food select, that is not the Select-option and moving them back into food_resource. The reason I only increment i if a resource is not copied is because the i:th item is always recalculated. So, as I remove one option the next option takes it place. If I were to increment i I would only access every other option. The same situation occure in the second while loop, where I pick the options I want in my food select.
When I move options back to the food select I check if the item has the selected attribute set and stor the index for that option in the variable selectedIndex. The reason for this is that javascript will by defalut select the last added element, regardles if any of them have the select attribute set or not. In the last line I manually change the selected item to the one that was actually seleted and if none of the options was selected it will select the first element.
To complete the functionallity I end by adding an event listender that calls the updateFood-function every time the category select is changed.
Hope this was usefull and if you want to try out the live code you can do that in my dependant select sample. If you have a cleaner solution than I'd love to see how you do it =)
- select, javascript, components, software